Prerequisites
- Products: Liquid UI WS, Liquid UI Server or Local DLL, Client Software
- Commands: pushbutton(), message(), _eventid
Purpose
Learn how to display custom popup windows with Yes/No and OK options using the reenter command with process functionality. You can include the required options in the popup window as per the requirement. Here, the eventid system variable captures the option selected on the popup windows. Based on the options selected on the popup window, the relevant process gets executed.
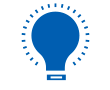
In this scenario, you will learn how to create a popup window and select the required option to act accordingly.
User Interface
//Create this file inside your script folder for customizing the SAP Easy Access screen: SAPLSMTR_NAVIGATION.E0100.sjs
//Now, let's start adding the Liquid UI script to the above file and save it.
- Logon to SAP and delete the image container using the del command on the SAP Easy Access screen, as shown below.
// Deletes an image container on the easy access screen
del("X[IMAGE_CONTAINER]");
- Add an input field with the label Sales Order, as shown in the image below.
//Creates an input field with Sales Order as the label name.
inputfield([2,2],"Sales Order",[2,20],{"name":"z_va02_order","size":18}); - Add a pushbutton with the label Change Order to execute the process ChangeOrder on the click.
//Creates a pushbutton with Change Order as label name and calls ChangeOrder the process on click. pushbutton([4,2], "@01@Change Order", "?", {"size":[2,70],"process":changeOrder});
- Now, add the following Liquid UI script to this file, and save it.
// Function prototype to trim blank characters from a string String.prototype.trim = function () { return this.replace(/^\s+|\s+$/g, ""); } // Function to return trimmed string function getString(strInput) { return (typeof(strInput) == 'undefined' || strInput == 'undefined') ? "" : strInput.toString().trim(); } // Function to check for blank string // Specifically used to determine if input exist in the edit field function isBlank(strInput) { var strVal = getString(strInput); var blank = strVal == ""; return blank; } // Function to delete toolbar buttons on popup function delButtonsRSM0400() { del('P[Continue]'); del('P[Generate]'); del('P[End Session]'); del('P[Cancel]'); if(<'P[Delete Session]'>.isValid) del("P[Delete Session]"); } // Function to display the message on '/o' popup with necessary formatting function procLUIMessageBox(param){ var intHeight = 5 + 4 + param.l_message.length; var intPaintRow = 1; var intPaintCol = 3; enter("/o"); onscreen 'RSM04000_ALV_NEW.2000' goto RESIZE_REPAINT; onscreen 'RSM04000_ALV.2000' RESIZE_REPAINT:; clearscreen(); delButtonsRSM0400(); title(param.l_title); if(isBlank(param.l_viewtext)) var viewTextContent=''; else var viewTextContent = param.l_viewtext; if(isBlank(param.l_viewhtml)) var viewHTMLContent=''; else var viewHTMLContent = param.l_viewhtml; if(isBlank(param.l_viewheight)) var viewHeight=0; else var viewHeight = param.l_viewheight; //Logic to render message for(var idxI=0; idxI<param.l_message.length; idxI++){ var intWidth = (intWidth>param.l_message[idxI].length)?intWidth:param.l_message[idxI].length; if(param.l_message[idxI].indexOf("\t")==0) //When message needs to be indented text([intPaintRow+idxI,intPaintCol+4], param.l_message[idxI]); else text([intPaintRow+idxI,intPaintCol], param.l_message[idxI]); } intWidth = intWidth+20; if(intWidth<51) intWidth=52; intPaintRow = 2 + param.l_message.length; if(!isBlank(fileURL)) { windowsize([5,5,90,intHeight+viewHeight]); view([intPaintRow-1,5],[intPaintRow+viewHeight,60],fileURL,{'name':fileURLName}); intPaintRow = intPaintRow+1; } else if(viewTextContent.length>0) { textbox([intPaintRow,1],[intPaintRow+14,148],{'name':'viewTextContent','readonly':true}); intPaintRow = intPaintRow+15; windowsize([5,5,150,intHeight+15]); } else if(viewHTMLContent.length>0) { var viewingFile = generatePrintableView(viewHTMLContent); var viewHNDL = getTodayDateTimeStr('HMS'); view([intPaintRow,1],[intPaintRow+14,146],viewingFile,{'name':viewHNDL}); intPaintRow = intPaintRow+15; windowsize([5,5,150,intHeight+15]); //removefile(viewingFile); } else { windowsize([5,5,intWidth,intHeight+1]); } fileURL = ''; //Draw buttons according to type switch(param.l_type) { case "MB_YESNO": pushbutton([intPaintRow,intPaintCol], "@01@Yes", "/12",{"eventid":"YES", "size":[2,20]}); pushbutton([intPaintRow,25], "@02@No", "/12",{"eventid":"NO", "size":[2,20]}); break; case "MB_OKCANCEL": pushbutton([intPaintRow,intPaintCol], "@01@OK", "/12",{"eventid":"OK", "size":[2,20]}); pushbutton([intPaintRow,25], "@02@Cancel", "/12",{"eventid":"CANCEL", "size":[2,20]}); break; case "MB_OK": pushbutton([intPaintRow+viewHeight,intPaintCol], "@01@OK", "/12",{"eventid":"OK", "size":[2,20]}); break; default: pushbutton([intPaintRow,intPaintCol], "OK", "/12",{"eventid":"OK", "size":[2,20]}); break; } } // Function to show how to call either 'MB_YESNO' or 'MB_OK' popup function changeOrder(){ if(isBlank(z_va02_order)){ message("E: Enter Order number"); enter('?'); goto SCRIPT_END; } onscreen "SAPLSMTR_NAVIGATION.0100" clearscreen(); tmp_msg = "Would you like to proceed \n to VA02 Change Order?"; strType = "MB_YESNO"; strTitle = "Information"; aryMessage = tmp_msg.split("\n"); tmp_msg = ""; reenter({"process":procLUIMessageBox, "using":{"l_type":strType, "l_title":strTitle, "l_message":aryMessage}}); onscreen "SAPLSMTR_NAVIGATION.0100" if(_eventid == 'YES'){ enter("/nVA02",{"process":navigateVA02}); } else { clearscreen(); tmp_msg = "Cancelled Process"; strType = "MB_OK"; strTitle = "Information"; aryMessage = tmp_msg.split("\n"); tmp_msg = ""; reenter({"process":procLUIMessageBox, "using":{"l_type":strType, "l_title":strTitle, "l_message":aryMessage}}); } onscreen "SAPLSMTR_NAVIGATION.0100" enter('?'); SCRIPT_END:; } // Function lands on VA02 and sets the Order number function navigateVA02(){ onscreen "SAPLSMTR_NAVIGATION.0100" enter("/nVA02"); onscreen "SAPMV45A.0102" set("F[Order]","&V[z_va02_order]"); enter('?'); }
- Open the SAP Easy Access screen and enter the Sales Order as 5235 number, as shown in the image below, and then, click the Change Order pushbutton.
- You can see a popup window with two options Yes/No, asking permission whether to Change Order.
- If you click Yes in the popup to proceed, you are navigated to the Change Sales Order screen with the order number displayed on the screen.
- If you click No in the popup window, you can view another popup stating that the process is canceled.
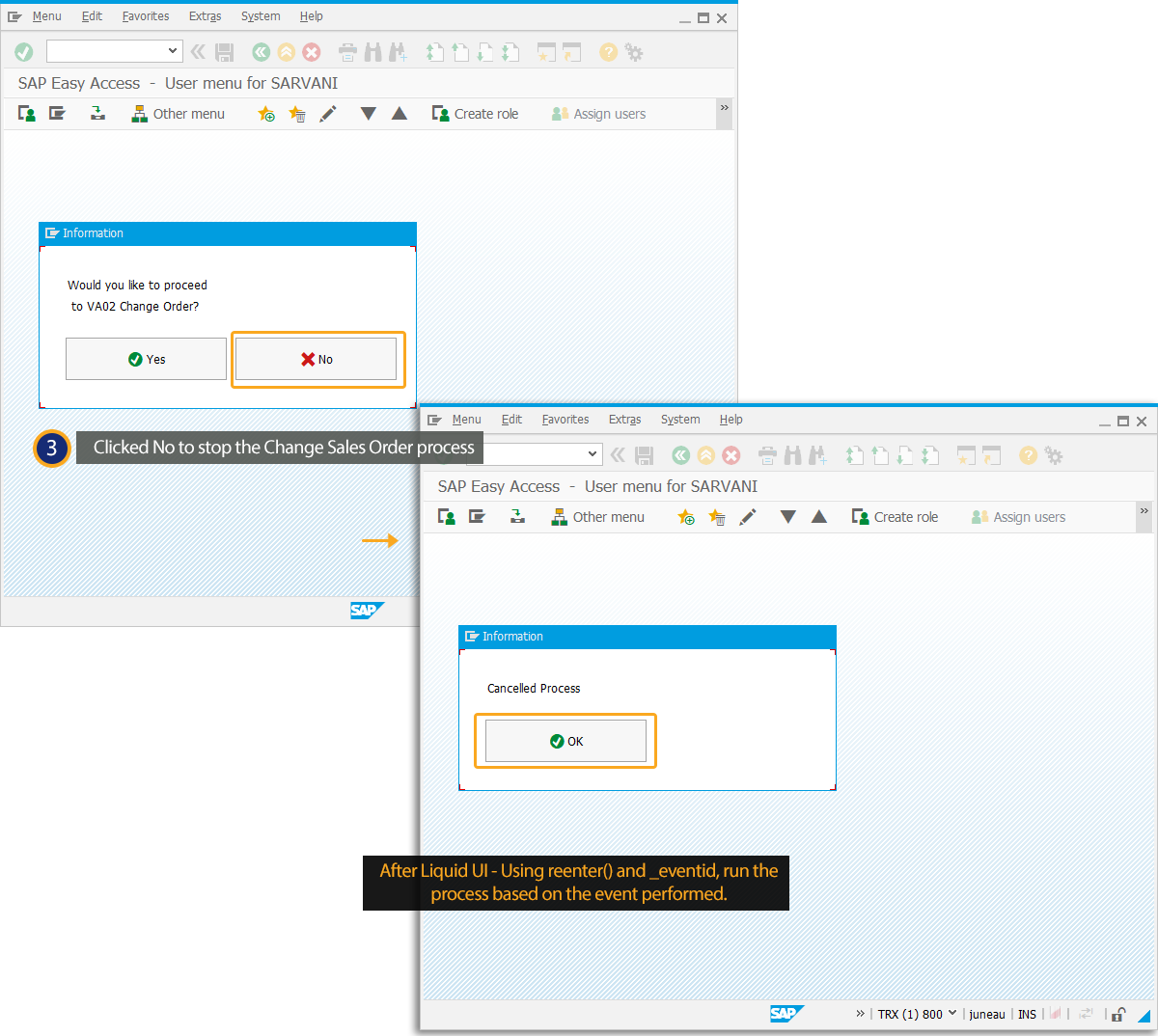