Prerequisites
- Products: Liquid UI WS, Liquid UI Server or Local DLL, Client Software
- Commands: load()
Purpose
You can learn how to perform accurate mathematical calculation with various decimal formats in SAP . To maintain compatibility in decimal notation during calculations in SAP, you can use the decimal conversion process to convert into specific decimal notation.
SAP Default Decimal Notation
SAP provides below decimal notation options for user profile default
- "X": 1,234,567.89
- "Y": 1 234 567,89
- " ": 1.234.567,89
User Interface
In this scenario you will learn how to change the decimal formats when Windows OS uses different decimal notation other than "1,234,567.89" for calculation.
To perform calculation, you need to convert the decimal format to raw format and then proceed. After completion of calculation, you can convert back to required SAP decimal format. You can view all the conversion steps explained in detail below:
Step 1: Create the function to convert specific format value to raw format
// Example: z_val = decimalNotationFormat("Y",Gweightitem.toString(),3); function decimalNotationFormat(numberFormat,number,nDec){ var str = ""; if(nDec == void 0) // Default for number of decimal places nDec = 2; switch(numberFormat) { case 'X': str = number.replace(/\,/g, ''); // Replace , with nothing break; case 'Y': str = number.replace(/\s+/g,''); // Remove Blank Spaces str = str.replace(/\,/g, '.'); // Replace , with . break; default: str = number.replace(/\./g, ''); // Replace . with nothing str = str.replace(/\,/g, '.'); // Replace , with . break; } if(str.indexOf(".")>-1){ if((str.length - (str.indexOf(".")+1)) > nDec) str = str.substring(0,(str.indexOf(".")+nDec+1)); } return str; }
Step 2: Create the function to convert raw format value to specific decimal notation format
// Example: z_value = userSAPDecimalFormat(z_val,"Y"); function userSAPDecimalFormat(nStr,nSeparator){ var fStr = nStr.toString().replace(/\,/g, ''); var str = fStr.split('.'); if(str[0].substring(0,1) == "-"){ var negative_val_flag = true; str[0] = str[0].substring(1,str[0].length); } else{ var negative_val_flag = false; } var offset = str[0].length % 3; if(nSeparator == ' ') str[0] = str[0].substring(0, offset) + str[0].substring(offset).replace(/([0-9]{3})/g, ".$1"); if(nSeparator == 'X') str[0] = str[0].substring(0, offset) + str[0].substring(offset).replace(/([0-9]{3})/g, ",$1"); if(nSeparator == 'Y') str[0] = str[0].substring(0, offset) + str[0].substring(offset).replace(/([0-9]{3})/g, " $1"); if(offset == 0) str[0] = str[0].substring(1,str[0].length); if(negative_val_flag) str[0] = "-" + str[0]; if(nSeparator == 'Y' || nSeparator == ' ') { return str.join(','); } else { return str.join('.'); } }
Step 3: Use above functions to build the calculation logic If Windows OS uses decimal notation setting as "1,234,567.89" but SAP requests"1 234 567,89":
mm01_mat_weight = decimalNotationFormat("Y", mm01_mat_weight.toString(), 3); //Convert material weight to raw format mm01_mat_qty = decimalNotationFormat("Y", mm01_mat_qty .toString(), 3); //Convert material quantity to raw format mm01_total_weight = parseFloat(mm01_mat_weight) * parseFloat(mm01_mat_qty); //Calculate material total quantity mm01_total_weight = userSAPDecimalFormat(mm01_total_weight, "Y"); //Convert material total quantity to SAP format "Y"
Step 4: If Windows OS doesn't use decimal notation as "1,234,567.89" If Windows OS uses decimal notation setting as "1 234 567,89"
mm01_total_weight = parseFloat(mm01_mat_weight.replace(/\s+/g,'')) * parseFloat(mm01_mat_qty.replace(/\s+/g,'')); //Calculate material total quantity mm01_total_weight = userSAPDecimalFormat(mm01_total_weight, "Y"); //Convert material total quantity to SAP format "Y" If Windows OS uses decimal notation setting as "1.234.567,89" mm01_total_weight = parseFloat(mm01_mat_weight.replace(/\./g,'')) * parseFloat(mm01_mat_qty.replace(/\./g,'')); //Calculate material total quantity mm01_total_weight = userSAPDecimalFormat(mm01_total_weight, " "); //Convert material total quantity to SAP format " "
Note: parseFloat() takes OS decimal notation format in order to convert, but it becomes raw format "1234567.89" after conversion
Next Steps
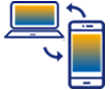
Learn how to display the value of radiobutton using .value property
10 min.
This article is part of the Javascript functions tutorial.