Prerequisites
- Products: Liquid UI WS, Liquid UI Server or Local DLL, Client Software
- Commands: pushbutton(), set(), inputfield(), comment(), text(), onscreen(), #include
Purpose
To provide a comprehensive overview of the process involved in integrating a file into the source file, leveraging the capabilities of the include preprocessor. Throughout the article, we will illustrate the incorporation of notification details from the Change Notification screen into the SAP Easy Access screen, guiding readers through the following steps:
- Add three required files to your script folder
- Delete unnecessary elements on the SAP Easy Access screen
- Add six input fields
- Add three push buttons to get the details
- Add a script file to attach files on the SAP screen
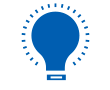
Note: This functionality is available from Liquid UI for SAP GUI Version 1.2.331 and Liquid UI Server Version 3.5.575.0. Ensure to avoid any spaces after the include statement to ensure it gets executed correctly.
User Interface
//Create this file inside your script folder for customizing the SAP Easy Access screen, SAPLSMTR_NAVIGATION.E0100.sjs
//Now, let's add the Liquid UI script to the above file and save it.
- Add the following files to your script folder
Add a file IW32_functions.sjs.
IW32_functions.sjs// Function prototype to trim blank characters from a string String.prototype.trim = function () { return this.replace(/^\s+|\s+$/g, ""); } // Function to return trimmed string function getString(strInput) { return (typeof(strInput) == 'undefined' || strInput == 'undefined') ? "" : strInput.toString().trim(); } // Function to check for blank string // Specifically used to determine if input exist in the edit field function isBlank(strInput) { var strVal = getString(strInput); var blank = strVal == ""; return blank; } // Clear Data function clearData(){ set("V[z*]",""); } // Get Order Information function getOrderInfo_1(){ if(isBlank(z_iw32_ordernum)){ return("E: Enter Order number"); } onscreen 'SAPLSMTR_NAVIGATION.0100' enter("/nIW32"); // Include Pre-processor makes the function inline #include "INC_GET_ORDER_DATA_1.sjs" onscreen 'SAPLCOIH.3000' set("V[z_iw32_salesorg]","&F[Sales Org.]"); set("V[z_iw32_distchannel]","&F[Distr. Channel]"); set("V[z_iw32_division]","&F[Division]"); enter("/n"); } // Get Order Information function getOrderInfo_2(){ #include "INC_GET_ORDER_DATA_2.sjs" }
Add INC_GET_ORDER_DATA_1.sjs file in your scripts folder.
INC_GET_ORDER_DATA_1.sjs// This function does error handling, calls include function and returns to main function // This function does error handling, calls include function and returns to main function // Include file with Syntax 1 onscreen 'SAPLCOIH.0101' set('F[Order]','&V[z_iw32_ordernum]'); enter(); onerror message(_message); enter("/n"); goto SCRIPT_END; onscreen 'SAPLCOIH.0101' if(_message.substring(0,2) == 'E:'){ message(_message); enter("/n"); goto SCRIPT_END; } else { enter("?"); } onscreen 'SAPLCOIH.3000' set("V[z_iw32_notifnum]","&F[Notifctn]"); set("V[z_iw32_funcloc]","&F[CAUFVD-TPLNR]"); //Func.Loc. set("V[z_iw32_funclocdesc]","&F[RIOT-PLTXT]"); //Func.Loc. Description enter("=ILOA"); SCRIPT_END:;
Add INC_GET_ORDER_DATA_2.sjs file in your scripts folder.
INC_GET_ORDER_DATA_2.sjs// Include file with Syntax 2 if(isBlank(z_iw32_ordernum)){ return("E: Enter Order number"); } onscreen 'SAPLSMTR_NAVIGATION.0100' enter("/nIW32"); onscreen 'SAPLCOIH.0101' set('F[Order]','&V[z_iw32_ordernum]'); enter(); onerror message(_message); enter("/n"); goto SCRIPT_END; onscreen 'SAPLCOIH.0101' if(_message.substring(0,2) == 'E:'){ message(_message); enter("/n"); goto SCRIPT_END; } else { enter("?"); } onscreen 'SAPLCOIH.3000' set("V[z_iw32_notifnum]","&F[Notifctn]"); set("V[z_iw32_funcloc]","&F[CAUFVD-TPLNR]"); //Func.Loc. set("V[z_iw32_funclocdesc]","&F[RIOT-PLTXT]"); //Func.Loc. Description enter("=ILOA"); onscreen 'SAPLCOIH.3000' set("V[z_iw32_salesorg]","&F[Sales Org.]"); set("V[z_iw32_distchannel]","&F[Distr. Channel]"); set("V[z_iw32_division]","&F[Division]"); enter("/n"); SCRIPT_END:;
- Delete unnecessary elements on the SAP Easy Access screen.
//Deletes unnecessary elements on the screen for(a=firstChild; a!=null; a=a.nextSibling) a.del();
- Add the Change Notification script file using the load command.
//Load a file into the script file load("IW32_functions.sjs");
- Add the required input fields to the screen, as shown below.
//Creates an input field labeled Order, with a technical name as z_iw32_ordernum and it shows as a mandatory field to enter value inputfield( [1,1], "Order", [1,18],{"name":"z_iw32_ordernum", "size":18, "required":true}); //Creates an inputfield with a label as Notification, technical name as z_iw32_notifnum inputfield( [5,1], "Notification", [5,18],{"name":"z_iw32_notifnum", "size":18}); //Creates an inputfield with a label as Func. Loc., technical name as z_iw32_funcloc inputfield( [6,1], "Func. Loc.", [6,18],{"name":"z_iw32_funcloc", "size":18}); //Creates an inputfield with a label as Sales Org., technical name as z_iw32_salesorg inputfield( [7,1], "Sales Org.", [7,18],{"name":"z_iw32_salesorg", "size":4}); //Creates an inputfield with a label as Distr. Channel, technical name as z_iw32_distchannel inputfield( [8,1], "Distr. Channel", [8,18],{"name":"z_iw32_distchannel", "size":2}); //Creates an inputfield with a label as Division, technical name as z_iw32_division and it shows as a mandatory field to enter value inputfield( [9,1], "Division", [9,18],{"name":"z_iw32_division", "size":2});
- Add three push buttons on the screen to run the required process.
//Creates a Clear fields pushbutton to run a clearData process on click. pushbutton([5,40],"Clear fields","?",{"process":clearData}); //Creates a Get Order Info [Syntax 1] pushbutton to run a getOrderInfo_1 process on click. pushbutton([2,1],"Get Order Info [Syntax 1]","?",{"process":getOrderInfo_1}); //Creates a Get Order Info [Syntax 2] pushbutton to run a getOrderInfo_2 process on click. pushbutton([3,1],"Get Order Info [Syntax 2]","?",{"process":getOrderInfo_2});
- Add comments to the push buttons to acknowledge the usage.
//Creates comments to display the usage of the push button. comment([2,30],"This function does error handling, calls include function and returns to main function [Check Script Syntax]"); comment([3,30],"The include function is being called entirely from the main function [Check Script Syntax]");
- Add a text beside the Func. loc. field to display its description.
//Creates text to display the value in the z_iw32_funclocdesc variable. text([6,38],"&V[z_iw32_funclocdesc]");
SAP Process
- Refresh the screen, enter the Order number, and click Get Order Info [Syntax 1] to display the necessary notification details in the respective fields. The function calls include functions, handle errors, and return to the main function.
- Click on Get Order Info [Syntax 2] to display the notification details in the respective fields. Here, the include function is being called entirely from the main function.
- Click the Clear fields push button to clear all the input fields on the Easy Access screen.
Next Steps
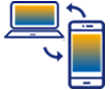
Learn how to retain your customized screen when opened in a new session
10 min.
This article is part of the Invoking functions tutorial.